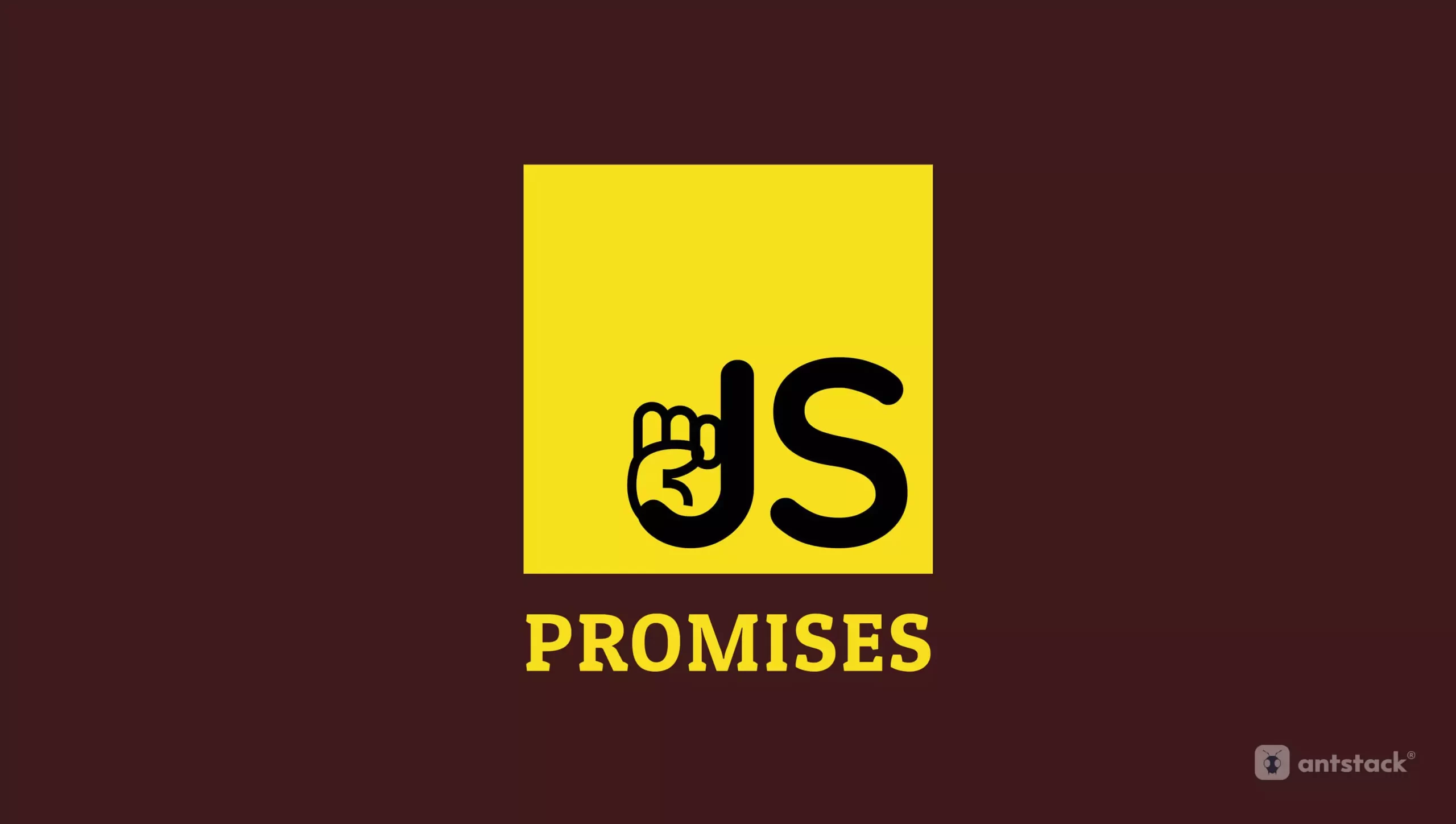
15 JavaScript Promise Interview Questions: A Complete Guide
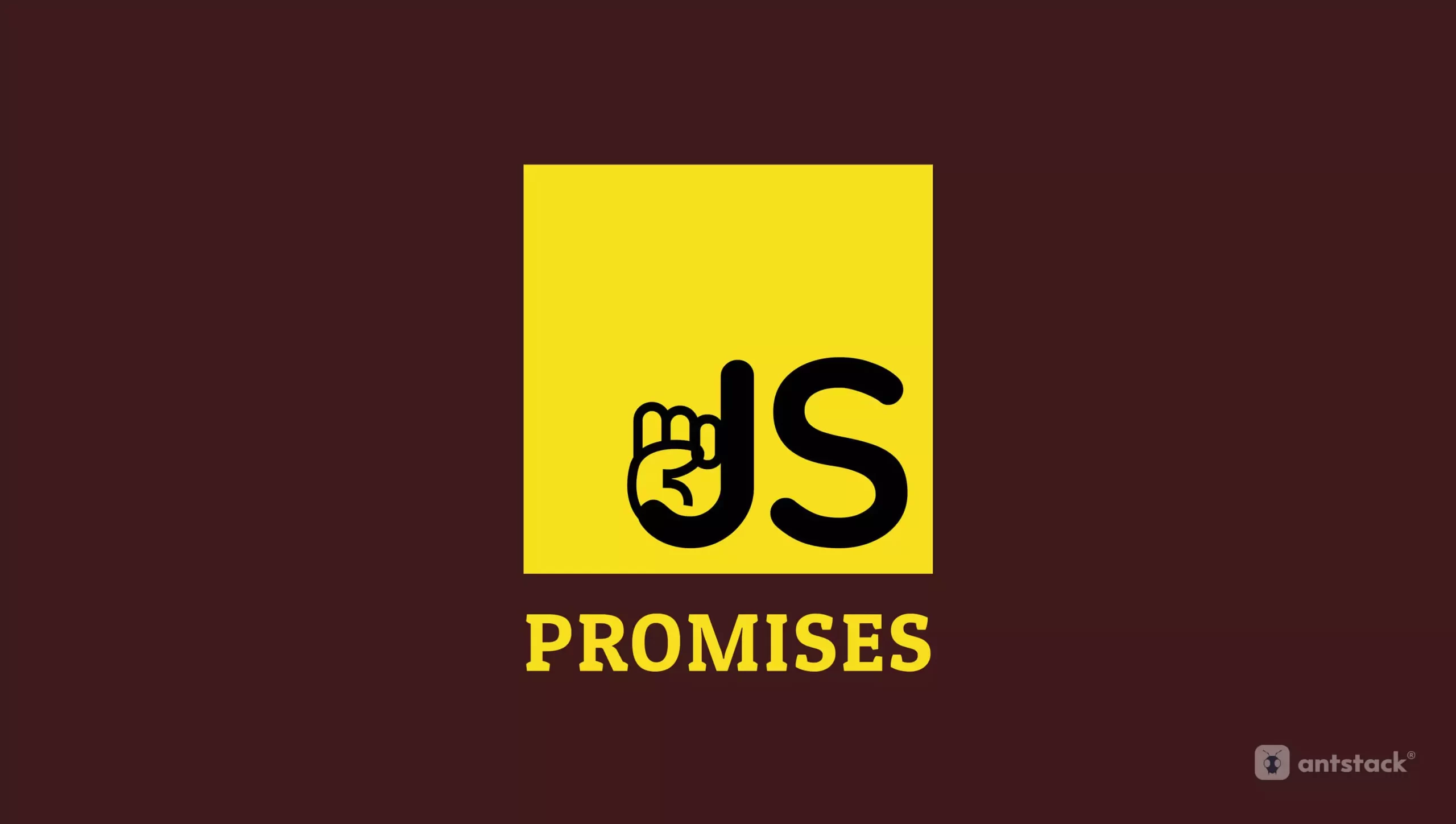
Promises are a basic notion in asynchronous programming in JavaScript, and it is essential to understand how to utilise them to write resilient and fast programmes. Promises provide a more flexible and maintainable method for handling asynchronous actions than previous callback-based techniques. With Promise-related questions, interviewers may assess a candidate’s mastery of JavaScript basics and their ability to build clean, legible code.
Top JavaScript Promise Interview Questions in 2023
JavaScript promises are a cornerstone of contemporary web development and may be found across the technology stacks of FANG (Facebook, Amazon, Netflix, and Google). As a result, it is common practise for these organisations to include promise-related interview questions. Promises are a crucial tool for managing the asynchronous processes at the heart of the high-performance, scalable web applications used by FANG businesses. To verify that applicants have a thorough knowledge of the issue and can develop fast and error-free code, interviewers may ask questions on promise chaining, error management, and the differences between promises and callbacks.
Frequently Asked Questions
As promises are widely utilised in today’s web development, prospective employers may prioritise applicants who are already acquainted with this technology. Without further ado, let’s dive into some crucial interview questions for the promise in javascript.
1. What is a JavaScript Promise?
A Promise is a JavaScript object that indicates the eventual success or failure of an asynchronous action, as well as its consequence. There are three possible states for a Promise: pending, fulfilled, or rejected.
2. How does Promise chaining operate and what is it?
Promise chaining is the process of stringing together numerous Promises using the then function. The then method produces a new Promise that is either fulfilled with the value returned by its fulfilment handler or rejected with the reason provided by its rejection handler. Here’s one instance:
fetch('/user')
.then(function(response) {
return response.json();
})
.then(function(user) {
console.log(user);
})
.catch(function(error) {
console.error(error);
});
This code retrieves a user from the server using the get method, parses the JSON response using the first then method, logs the user to the console using the second then method, and detects any problems using the catch method. Each then method produces a new Promise that is used to connect subsequent Promises.
3. How is a Promise created in JavaScript?
Promises can be created using the Promise function Object() { [native code] }. Promise’s function Object() { [native code] } accepts a function with two arguments: resolve and refuse. Resolve is invoked after the Promise has been fulfilled, whereas reject is invoked when the Promise has been denied.
4. How do you manage mistakes in a JavaScript Promise chain?
The catch method may be used to manage mistakes inside a Promise chain. The catch method is invoked if any of the chain’s Promises are refused.
5. What is the difference between JavaScript’s Promise.all and Promise.race?
Promise.all waits for all Promises in an array to be completed, while Promise.race delivers the outcome of the first Promise to be fulfilled or rejected.
6. What differentiates a resolved Promise from a rejected Promise in JavaScript?
A resolved Promise has finished successfully and has a result value. A rejected Promise has met an error and is accompanied by an explanation for the issue.
7. How does JavaScript wait for several Promises to complete?
Promise.all may be used to wait for numerous Promises to fulfil. Promise.all accepts an array of Promises and returns a Promise that is fulfilled when all of the array’s Promises have been satisfied.
8. How do you manage mistakes in a JavaScript Promise chain?
The catch method may be used to manage mistakes inside a Promise chain. The catch method is invoked if any of the chain’s Promises are refused.
9. How does JavaScript wait for several Promises to complete?
Promise.all may be used to wait for numerous Promises to fulfil. Promise.all accepts an array of Promises and returns a Promise that is fulfilled when all of the array’s Promises have been satisfied.
10. How do you build a Promise in JavaScript that resolves after a specified length of time?
With setTimeout and a Promise, you may build a Promise that resolves after a specified length of time. The setTimeout function is used to postpone the resolve function’s execution.
function delay(time) {
return new Promise(function(resolve) {
setTimeout(resolve, time);
});
}
delay(1000).then(function() {
console.log('Promise resolved after 1 second');
});
This generates a Promise that resolves after one second, following which a message is logged to the console.
11. How does JavaScript cancel a Promise?
With JavaScript, there is no native mechanism to cancel a Promise. Instead, you may use a flag to indicate that the Promise should be cancelled and then check for the flag inside the Promise method.
12. How are Promises chained in JavaScript?
Using the then function in JavaScript, you may chain Promises together. The then method accepts two functions as arguments: one to handle the fulfilment of the Promise and another to handle its rejection. Here’s one instance:
function fetchUser() {
return fetch('/user').then(function(response) {
return response.json();
});
}
function displayUser() {
fetchUser().then(function(user) {
console.log(user);
});
}
displayUser();
This code retrieves a user from a server using the get method, parses the JSON response, and logs the user to the console. The fetchUser function produces a Promise that resolves with the parsed JSON response, and the displayUser function attaches a then method to this Promise in order to log the user to the console.
13. How do you utilise Promises with async/await in JavaScript?
Async/await is a syntax sugar that makes dealing with Promises easier. You can build an asynchronous function that returns a Promise using the async keyword, and you can wait for a Promise to resolve using the await keyword. Here’s one instance:
async function fetchUser() {
const response = await fetch('/user');
const user = await response.json();
return user;
}
async function displayUser() {
const user = await fetchUser();
console.log(user);
}
displayUser();
This code is identical to the preceding example, which utilised Promises with the then function, but instead utilises async/await. The fetchUser method provides a Promise that resolves with the JSON response, and the displayUser function utilises await to wait for this Promise to resolve prior to logging the user to the console.
14. How do you build a Promise in JavaScript that never resolves?
By returning a Promise that is never fulfilled or refused, you might build a Promise that never resolves. Here’s one instance:
function neverResolve() {
return new Promise(function() {});
}
neverResolve().then(function() {
console.log('Promise resolved');
}).catch(function() {
console.log('Promise rejected');
}).finally(function() {
console.log('Promise finished');
});
This code generates an unresolved Promise and reports messages to the console when the Promise is resolved, rejected, or completed using the finally function.
15. What is JavaScript’s Promise.allSettled and how does it function?
Promise.allSettled is a new ES2020 method that returns a fulfilled Promise with an array of objects indicating the fulfilment and rejection values of the input Promises. Promise.allSettled, unlike Promise.all, waits for all Promises to settle, regardless of whether they were fulfilled or refused. Here’s one instance:
Promise.allSettled([
Promise.resolve('fulfilled'),
Promise.reject(new Error('rejected'))
])
.then(function(results) {
console.log(results);
});
This code generates a pair of Promises, one of which is fulfilled with the string “fulfilled” and the other of which is refused with an error. The Promise.allSettled function provides a fulfilled Promise containing an array of two objects that reflect the fulfilment and rejection values of the input Promises. The results array appears as follows:
[
{ status: 'fulfilled', value: 'fulfilled' },
{ status: 'rejected', reason: Error('rejected') }
]
• • •
Candidates that demonstrate a mastery of JavaScript promises are more likely to succeed in gaining a position at a FANG company. Candidates may exhibit their expertise in promise-related topics by studying and practising them extensively in order to demonstrate their ability to develop high-performance, scalable, and error-free code.
Candidates may maintain their competitive edge in the job market by keeping up with the newest programming advancements and information. Subscribe to our newsletter if you’re interested in keeping up with our programming and receiving frequent updates and material. Keep Coding!!!